What is CVV? The Card Verification Value (CVV) is an extra code printed on your debit or credit card. It sometimes called Card Verification Value (CVV or CV2), Card Verification Value Code (CVVC), Card Verification Code (CVC), Verification Code (V-Code or VCode), or Card Code Verification (CCV). CVV is a new authentication procedure established by credit card companies to further efforts towards reducing fraud for internet transactions. It consists of requiring a card holder to enter the CVV number in at transaction time to verify that the card is on hand.
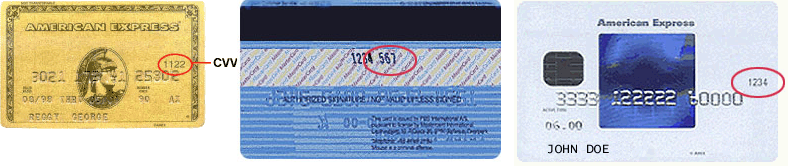
CVV for Visa, MasterCard, BankCard and Diners is the final three digits of the number printed on the signature strip on the back of your card. CVV for American Express appears as a separate 4-digit code printed on the front of your card.CVV Code ValidationWe will see how to validate a CVV number using JavaScript as well as C# in this article. I have written methods/functions in C# and JavasScript respectively for this validation. The JavaScript function validateCvvCode() is used to validate a CVV code at client side. The ValidateCVVCode() written in C# is used to check the CVV code at server side. These functions determines number of digits required for the given card type and then checks if the CVV Code have the required count of digits and have only numeric digits 0 to 9 using a regular expression. The regular expression is formed dynamically based on the card type.
Validation Using JavaScript The following code contains the javascript function to validation CVV code with HTML markup of the demo application.
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="CVVValidationDemo.aspx.cs" Inherits="CVVValidationDemo" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title>CVV Credit Card Number Validation </title> <script type="text/javascript" language="javascript">
function validateCvvCode() {
//Get the text of the selected card type var cardType = document.getElementById('ddlCardType') .options[document.getElementById('ddlCardType').selectedIndex].text; // Get the value of the CVV code var cvvCode = document.getElementById('txtCVVCode').value;
var digits = 0; switch (cardType.toUpperCase()) { case 'MASTERCARD': case 'EUROCARD': case 'EUROCARD/MASTERCARD': case 'VISA': case 'DISCOVER': digits = 3; break; case 'AMEX': case 'AMERICANEXPRESS': case 'AMERICAN EXPRESS': digits = 4; break; default: return false; }
var regExp = new RegExp('[0-9]{' + digits + '}'); return (cvvCode.length == digits && regExp.test(cvvCode)) }
function checkCvvCode() {
var result = validateCvvCode(); if (result) alert('Valid CVV Code'); else alert('Invalid CVV Code'); }
</script>
<style type="text/css"> .style1 { font-size: large; font-weight: bold; font-family: Arial; } </style> </head> <body> <form id="form1" runat="server"> <div> <table width="100%" cellspacing="0" cellpadding="0"> <tr> <td width="200px"> </td> <td class="style1"> CVV Code Validation </td> </tr> <tr> <td width="200px"> </td> <td> <asp:ValidationSummary ID="vsCVVValidationSummary" runat="server" /> </td> </tr> <tr> <td width="200px"> Card Type </td> <td> <asp:DropDownList ID="ddlCardType" runat="server" Width="250px"> </asp:DropDownList> </td> </tr> <tr> <td width="200px"> </td> <td> </td> </tr> <tr> <td width="200px"> CVV Code </td> <td> <asp:TextBox ID="txtCVVCode" runat="server"></asp:TextBox> <asp:CustomValidator ID="custValidCVV" runat="server" ErrorMessage="Invalid CVV Code">*</asp:CustomValidator> </td> </tr> <tr> <td width="200px"> </td> <td> </td> </tr> <tr> <td width="200px"> </td> <td> <asp:Button ID="btnCvvServerValidate" runat="server" Text="Server Side Validation" OnClick="btnCvvServerValidate_Click" /> <input id="hbtnCvvClientValidate" type="button" value="Client Side Validation" onclick="checkCvvCode()" /> </td> </tr> </table> </div> </form> </body> </html>
Validation Using C#
The following code snippet has server side written in C# to demonstrate the CVV code validation. I have loaded and checked the following card types in a dropdown list for demonstration purpose: MASTERCARD, EUROCARD, EUROCARD/MASTERCARD, VISA, DISCOVER and AMERICAN EXPRESS.
using System; using System.Data; using System.Text.RegularExpressions;
public partial class CVVValidationDemo : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) BindCardType(); }
private void BindCardType() { System.Data.DataTable dtCardType = new System.Data.DataTable(); dtCardType.Columns.Add(new DataColumn("Card_Type_Id", typeof(int))); dtCardType.Columns.Add(new DataColumn("Card_Type_Name", typeof(string)));
dtCardType.Rows.Add(new object[] { 1, "MASTERCARD" }); dtCardType.Rows.Add(new object[] { 2, "EUROCARD" }); dtCardType.Rows.Add(new object[] { 3, "EUROCARD/MASTERCARD" }); dtCardType.Rows.Add(new object[] { 4, "VISA" }); dtCardType.Rows.Add(new object[] { 5, "DISCOVER" }); dtCardType.Rows.Add(new object[] { 6, "AMEX" }); dtCardType.Rows.Add(new object[] { 7, "AMERICANEXPRESS" }); dtCardType.Rows.Add(new object[] { 8, "AMERICAN EXPRESS" });
ddlCardType.DataSource = dtCardType; ddlCardType.DataValueField = "Card_Type_Id"; ddlCardType.DataTextField = "Card_Type_Name"; ddlCardType.DataBind();
} protected void btnCvvServerValidate_Click(object sender, EventArgs e) { custValidCVV.ErrorMessage = "Invalid CVV Code"; custValidCVV.IsValid = ValidateCVVCode(); }
private bool ValidateCVVCode() { var cardType = ddlCardType.SelectedItem.Text; var cvvCode = txtCVVCode.Text;
var digits = 0; switch (cardType.ToUpper()) { case "MASTERCARD": case "EUROCARD": case "EUROCARD/MASTERCARD": case "VISA": case "DISCOVER": digits = 3; break; case "AMEX": case "AMERICANEXPRESS": case "AMERICAN EXPRESS": digits = 4; break; default: return false; }
Regex regEx = new Regex("[0-9]{" + digits + "}"); return (cvvCode.Length == digits && regEx.Match(cvvCode).Success); } }
|
0 comments:
Post a Comment